03/08/2023
If you are a developer, you are used to VS Code or other tools where you write your code. Like me, I'm sure you configured everything the way you like it. From autosuggestion to the right syntax highlighting style and color. In that case you will want to write your Custom Coded Action locally then push it to HubSpot. In this blog article, I will explain to you how to do so.
What HubSpot gives us by default
On the platform in a Workflow, if you add a Custom Coded Action block, you have an interface where you can select the programming language you would like to use. A dropdown to manage / use your API secrets etc…
Underneath, you can write your code, but in that small editor there’s no autocompletion, you can’t format automatically your code. You can’t automatically write doc blocks for your functions…
That’s why I prefer to write my code locally in my IDE, that the way I produce better code, and I can also keep track of my changes with Git.
If you are interested to set up something similar, I will explain in this article how to set up a Custom Coded Action enviroment locally.
A JavaScript CLI to re-create the environment
The main idea is to have a local environment which provides the same functions and limitation as HubSpot. That’s why I created a CLI where I re-created the functions which are unique to the HubSpot Custom Coded Actions.
With this tool you can use your own IDE and feel like home.
prerequisites
- JavaScript / Node.js / Git knowledge
- Git
- Node.js installed on your machine
Install the CLI
To install the CLI clone the following repository :
git clone https://github.com/Antoinebr/HubSpot-Operations-Hub-Custom-Coded-Action.git
Then install all the dependencies
cd HubSpot-Operations-Hub-Custom-Coded-Action
npm install
Start a new project
To start a new project type :
npm run init my-new-custom-coded-action
This will copy the folder __TEMPLATE__
which is a scaffolding of a Custom Coded Action
Understand the unique functions and variables
In this video, I will explain some specific functions and variable used in the Custom Coded Actions. Nothing complicated, but still very important.
Manage the secrets in HubSpot
In a Custom Coded Action you can manage your secrets, which is ideal to store an API key for example. Indeed, you do not want to have your API keys displayed in your code, you will want to have them as environment variable.
To create a new secret :
To access you secret use :
process.env.mySuperAPIKey;
console.log(process.env.mySuperAPIKey);
Use the contact, company… properties in the Custom Coded Action
To access the properties, first go to the section Property to include in code
. In that section, you can use any property of the current object, and you can choose the variable name you want.
In that example, we can access the contact’s email by using :
event.inputFields.email
Get the id of the current object
If you need the id of the current object enrolled in the WorkFlow you can use :
event.object.objectId
Run your code locally
Manage / access the secrets locally
To use a secret locally, add it to your .env
file at the root the cli project.
Set your secret in the .env
like so
privateAppToken = "vcf-no9-ggt09tnz-a522-4002-b012-jngpmsecx"
dropContactAPI= "qdkqsnksqnknvvvppmqmqsdds"
To access the secret in your code, in the cca.js
where you write your code use :
process.env.privateAppToken
Watch the video to learn more :
Manage / access the objects’ properties locally
To set a property to include in code locally, add to the ./your-cca/event.js
file the keys you need :
exports.events = {
object: {
objectId: 3401
},
inputFields: {
domainName : "google.com",
email : "hello@gmail.com"
}
}
To access it in your code
const domainName = event.inputFields.domainName;
const email = event.inputFields.email;
console.log('--> ', domainName, '-----> ', email)
Manage / access the current object’s id
If your code, uses the object’s ID of the current object in the workflow you can use.
event.object.objectId; // this returns the id it the current object
To set it up locally in the « event.js« ` update the value :
exports.events = {
object: {
objectId: 3401
},
inputFields: {
domainName : "google.com",
email : "hello@gmail.com"
}
}
Test the code
To test the code type in your terminal :
npm run cca ./your-cca/cca.js
This runs your code and display what will be returned by the Custom Coded Action from the output function.
As you can see :
This very basic Custom Coded Action :
exports.main = async (event, callback) => {
const domainName = event.inputFields.domainName;
const email = event.inputFields.email;
callback({
outputFields: {
domainName,
email
}
});
}
Will display this in your terminal :
Deploy it
To deploy it nothing easier, copy the cca.js and paste in HubSpot.
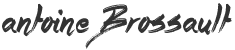