23/01/2024
Within the Operations Hub, there exists a powerful feature known as Custom Coded Actions, which are essentially server-side functions executable within workflows.
These code blocks or functions can be written in either JavaScript or Python. However, a significant challenge arises as not everyone possesses proficiency in these programming languages. Fortunately, with the advent of AI technologies like ChatGPT, generating code becomes feasible by providing appropriate prompts.
This article aims to guide you through the process of generating a Custom Coded Action using minimal code intervention. We’ll explore specific prompts that enable the creation of these actions without delving too deep into coding intricacies.
Disclaimer :
It’s crucial to note that while AI can generate code snippets, utilizing them effectively requires a basic understanding of coding principles. Even if an AI can generate the code, comprehending and making necessary adjustments can be challenging without some level of coding knowledge. Therefore, this article will not only showcase the generation process but also underscore the importance of comprehending and refining the code for optimal usage.
The prompt :
I’ve crafted a prompt for you—it’s a fundamental one that can be tailored to your specific requirements. While I’ve conducted some testing and found it to be effective for a basic start, feel free to refine it further based on your needs.
To provide a quick overview, this prompt outlines the constraints associated with a Custom Coded Action. If you’re unfamiliar with these constraints, I strongly recommend reading my article titled « How to Write Your First Custom Coded Action » for a comprehensive understanding.
Now, let’s delve into the prompt:
Context : serverless function developement
Help Needed : I want you to help me to write a logic with JavaScript
Constrains : the code should we written in this format :
exports.main = async (event, callback) => {
// code here
callback({
outputFields: {
}
});
}
The logic should be written inside the main function.
If the logic returns data the data should be returned by the callback function inside the outputFields object.
When you get external data input as parameters. The data comes from event.inputFields object. E.g : you get the contact email adress then the email variable should be set like :
const email = event.inputFields.email;
If you need to make HTTP request you can use the axios library.
logic to implement :
Copy the provided prompt into the ChatGPT interface and articulate your logic as you would in a coding environment. Break down your thought process into individual steps, considering each step from a coder’s perspective.
Once you’ve input the prompt, articulate your logic methodically, focusing on clarity and precision in each step. Think of it as a conversation with the AI where you guide it through your logical reasoning, ensuring a comprehensive understanding of the desired outcome.
Remember, effective communication is key, so express your logic in a way that facilitates a smooth and accurate generation of the Custom Coded Action.
Demo : detect if an email is from a free email provider
In this demonstration, I will guide you through the process of creating a Custom Coded Action that determines whether an email address is associated with a free email provider or not.
For example, addresses like antoine@gmail.com are considered free, as they can be created by anyone using services like Gmail, Yahoo, Hotmail, and so forth.
Contrastingly, an email address such as: antoine@hubspot.com would fall into a different category.
Here’s what I added to the prompt :
I want you to grab the contact email address and tell me if the domain is a free one
Like : antoine@gmail.com is a free one antoine@HubSpot.com is not a free one
Let see what chat GPT generated :
const isFreeDomain = (email) => {
// List of free email domains
const freeDomains = ['gmail.com', 'yahoo.com', 'outlook.com'];
// Extract the domain from the email address
const [, domain] = email.split('@');
// Check if the domain is in the list of free domains
return freeDomains.includes(domain.toLowerCase());
};
exports.main = async (event, callback) => {
// Get the contact email address from the inputFields
const email = event.inputFields.email;
// Check if the domain is a free one
const isFree = isFreeDomain(email);
// Prepare the outputFields object to be returned by the callback
const outputFields = {
isFreeDomain: isFree,
};
// Call the callback function with the outputFields
callback({
outputFields,
});
};
Now the only thing to make it a bit better would be to ask chat GPT to add more free domains.
Let’s do it :
Ok this is what I have now :
const isFreeDomain = (email) => {
// List of free email domains
const freeDomains = [
'gmail.com', 'yahoo.com', 'outlook.com', 'hotmail.com', 'aol.com', 'protonmail.com',
'icloud.com', 'zoho.com', 'mail.com', 'gmx.com', 'yandex.com', 'mail.ru',
'inbox.com', 'fastmail.com', 'tutanota.com', 'cock.li', 'tuta.io', 'tuta.io',
'runbox.com', 'disroot.org', 'riseup.net', 'startmail.com', 'vfemail.net',
'countermail.com', 'safe-mail.net', 'mailinator.com', 'guerrillamail.com',
'10minutemail.com', 'temp-mail.org', 'anonbox.net', 'mailnesia.com'
];
// Extract the domain from the email address
const [, domain] = email.split('@');
// Check if the domain is in the list of free domains
return freeDomains.includes(domain.toLowerCase());
};
exports.main = async (event, callback) => {
// Get the contact email address from the inputFields
const email = event.inputFields.email;
// Check if the domain is a free one
const isFree = isFreeDomain(email);
// Prepare the outputFields object to be returned by the callback
const outputFields = {
isFreeDomain: isFree,
};
// Call the callback function with the outputFields
callback({
outputFields,
});
};
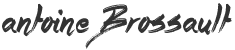